Connect your app to Azure Cosmos DB Emulator on Docker. PART 1
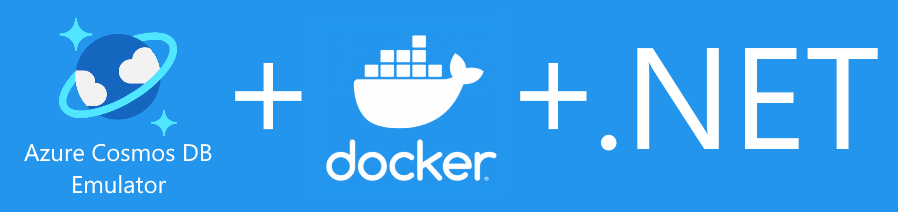
Recently I’ve tried to use official Cosmos DB Emulator on docker , but quickly encountered some issues that I’ll try to address in this article.
Following documentation I’ve managed to run emulator on Docker with no problem, but issues start appearing when I’ve tied to connect my application to it. Basically, I’ve had application that also running on Docker, but unfortunately documentation does not cover this scenario. So, to figure our how to make it work I’ve decided to run my application without Docker, just to make sure that everything is working, but again there were some small issues.
In this article I’ll cover two scenarios:
- Connect to simulator from application on the local machine (PART 1)
- Connect to simulator from application that also on Docker (PART 2)
Run simulator on Docker
Lets start from running simulator first.
-
Create
docker-compose.yml
file -
Add following content
version: '3.4' services: azure.cosmosdb: image: mcr.microsoft.com/cosmosdb/linux/azure-cosmos-emulator container_name: azure.cosmosdb environment: - AZURE_COSMOS_EMULATOR_PARTITION_COUNT=5 - AZURE_COSMOS_EMULATOR_ENABLE_DATA_PERSISTENCE=true - AZURE_COSMOS_EMULATOR_IP_ADDRESS_OVERRIDE=x.x.x.x ports: - 8081:8081 - 10251:10251 - 10252:10252 - 10253:10253 - 10254:10254
-
Set
AZURE_COSMOS_EMULATOR_IP_ADDRESS_OVERRIDE
variable to IP address of your local machine. To get you IP address run following command:On Windows
ipconfig
On Linux or MacOS
ifconfig | grep "inet " | grep -Fv 127.0.0.1 | awk '{print $2}' | head -n 1
NOTE: this variable is important if you want to connect to emulator from your local machine. That is where I had one of my issues, because I thought it is not needed.
-
Now you can run emulator
docker-compose up -d -f <you compose file>
or you if prefer to use docker directly
docker run -d --name azure.cosmosdb -e AZURE_COSMOS_EMULATOR_PARTITION_COUNT=5 -e AZURE_COSMOS_EMULATOR_ENABLE_DATA_PERSISTENCE=true -e AZURE_COSMOS_EMULATOR_IP_ADDRESS_OVERRIDE=<YOUR IP ADDRESS> -p 8081:8081 -p 10251:10251 -p 10252:10252 -p 10253:10253 -p 10254:10254 mcr.microsoft.com/cosmosdb/linux/azure-cosmos-emulator
Install Certificate
After simulator is running you need to download simulator self-signed certificate and install it to you machine trusted store.
-
To download certificate just open in the browser following https://localhost:8081/_explorer/emulator.pem link and save the certificate file, but make sure you gave it
.crt
extension. Or you can run following command:curl -k https://localhost:8081/_explorer/emulator.pem > azure.cosmosdb.crt
NOTE: if you open this link in your browser it will show you a warning that you are trying to access suspicious site, which is expected because we did not installed certificate yet.
-
Because this is a self-signed certificate we need to add it to our trusted certificates.
On Windows
- Double click on certificate.
- Click Install certificate….
- Select Current User and click Next.
- IMPORTANT: Select Place all certificates in the following store and click Browse. Because by default it installs into Personal stores. And most likely certificate validation in you application will not work out of the box.
- Select Trusted Root Certification Authority and click Ok, then Next and Finish.
- When Security Warning window appears click Yes button.
On Linux
-
Copy certificate to the following folder
/usr/local/share/ca-certificates
sudo cp azure.cosmosdb.crt /usr/local/share/ca-certificates/azure.cosmosdb.crt
-
Update certificate authority
sudo update-ca-certificates
On MacOS
- Open the Keychain Access app on your Mac to import the emulator certificate.
- Select File and Import Items and import the
azure.cosmosdb.crt
. - After the
azure.cosmosdb.crt
is loaded into KeyChain, double-click on the localhost name and change the trust settings to Always Trust.
-
After certificate was installed you may test it by navigating to the emulator portal using following link https://localhost:8081/_explorer/index.html . If certificate was installed correctly you should not see any warnings
Connect Application on Local Machine
Now lets try to connect our application to the emulator.
-
In Visual Studio create .NET 6 console application or simply run following command:
dotnet new console --name CosmosDbTest
-
To the
*.csproj
file add nuget reference to the EntityFramework<ItemGroup> <PackageReference Include="Microsoft.EntityFrameworkCore.Cosmos" Version="6.0.0" /> </ItemGroup>
-
Add following code to the
Program.cs
fileusing Microsoft.EntityFrameworkCore; using Microsoft.Extensions.DependencyInjection; var context = new ServiceCollection() .AddCosmos<CosmosDbContext>( connectionString: "AccountEndpoint=https://localhost:8081/;AccountKey=C2y6yDjf5/R+ob0N8A7Cgv30VRDJIWEHLM+4QDU5DE2nQ9nDuVTqobD4b8mGGyPMbIZnqyMsEcaGQy67XIw/Jw==", databaseName: "CosmosDb") .BuildServiceProvider() .GetRequiredService<CosmosDbContext>(); await context.Database.EnsureCreatedAsync(); context.Entity.Add(new Entity(Guid.NewGuid().ToString())); await context.SaveChangesAsync(); class CosmosDbContext : DbContext { public CosmosDbContext(DbContextOptions<CosmosDbContext> options) : base(options) { } public DbSet<Entity> Entity { get; set; } = null!; } public record Entity(string Id);
NOTE: connection string you can find on the main page of the emulator portal
-
Hit
F5
. Application should run without any issues. -
Check result on the emulator portal
Possible Issues
If everything was done correctly you should not have any issues. But when you run your application you may see following issues:
The remote certificate is invalid because of errors in the certificate chain: UntrustedRoot
To resolve this issue, make sure you properly has installed the certificate into the Trusted Root store.
The I/O operation has been aborted because of either a thread exit or an application request
If you see this error, make sure that you has set AZURE_COSMOS_EMULATOR_IP_ADDRESS_OVERRIDE
variable with correct IP address of your local machine
Source Code
The sample application for this article you can find here .